Flask๋ ํ ํ๋ฆฟ์ ์ฌ์ฉํ์ฌ ์น ํ์ด์ง๋ฅผ ๋์ ์ผ๋ก ๋ ๋๋งํ ์ ์๋ค.
ํ ํ๋ฆฟ์ HTML ํ์ผ๊ณผ ์ ์ฌํ์ง๋ง ๊ทธ ์์์ ํ์ด์ฌ ์ฝ๋(Jinja2 ํ ํ๋ฆฟ ์ธ์ด)๋ฅผ ์ฌ์ฉํ์ฌ ๋ฐ์ดํฐ๋ฅผ ๋์ ์ผ๋ก ์ฝ์ ํ๊ณ ์ ์ด
1. ํ ํ๋ฆฟ ํ์ผ ๋๋ ํ ๋ฆฌ ๊ตฌ์กฐ
/project_name
|-- app.py
|-- config.py
|-- models.py
|-- templates/
|-- categories.html
|-- products.html
|-- users.html
|-- test.db
templates/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page</h1>
<p>Click below to see the categories, products, or users:</p>
<ul>
<li><a href="/get_categories">View Categories</a></li>
<li><a href="/get_products">View Products</a></li>
<li><a href="/get_users">View Users</a></li>
</ul>
</body>
</html>
templates/categories.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Categories</title>
</head>
<body>
<h1>Categories</h1>
{% if categories %}
<ul>
{% for category in categories %}
<li>{{ category.name }}</li>
{% endfor %}
</ul>
{% else %}
<p>No categories available.</p>
{% endif %}
</body>
</html>
templates/products.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Products</title>
</head>
<body>
<h1>Products</h1>
{% if products %}
<ul>
{% for product in products %}
<li>{{ product.name }} - ${{ product.price }} (Category: {{ product.category.name }})</li>
{% endfor %}
</ul>
{% else %}
<p>No products available.</p>
{% endif %}
</body>
</html>
templates/users.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Users</title>
</head>
<body>
<h1>Users</h1>
{% if users %}
<ul>
{% for user in users %}
<li>{{ user.username }} - {{ user.email }}</li>
{% endfor %}
</ul>
{% else %}
<p>No users available.</p>
{% endif %}
</body>
</html>
app.py
from flask import Flask, jsonify, render_template
from models import db, Product, User, Category
import config
from flask_migrate import Migrate
app = Flask(__name__)
app.config.from_object(config)
# ORM ์ด๊ธฐํ
db.init_app(app)
# Flask-Migrate ์ด๊ธฐํ
migrate = Migrate(app, db)
@app.route('/')
def index():
# ๊ธฐ๋ณธ ๋ฉ์ธ ํ์ด์ง ๋ ๋๋ง
return render_template('index.html')
@app.route('/add_category')
def add_category():
# ์๋ก์ด Category ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
new_category = Category(name="Electronics")
db.session.add(new_category)
db.session.commit()
return "Category added!"
@app.route('/add_product')
def add_product():
# ์๋ก์ด Product ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
# ์ด๋ฏธ ์กด์ฌํ๋ Category ID๋ฅผ ํ ๋นํ์ฌ Product์ ์ฐ๊ฒฐ
category = Category.query.first() # ์ฒซ ๋ฒ์งธ ์นดํ
๊ณ ๋ฆฌ ๊ฐ์ ธ์ค๊ธฐ (์์๋ก)
new_product = Product(name="Laptop", price=999.99, category_id=category.id)
db.session.add(new_product)
db.session.commit()
return "Product added!"
@app.route('/add_user')
def add_user():
# ์๋ก์ด User ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
new_user = User(username="sample_user", email="user@example.com")
db.session.add(new_user)
db.session.commit()
return "User added!"
@app.route('/get_categories')
def get_categories():
# DB์์ ๋ชจ๋ Category ์กฐํ
categories = Category.query.all()
return render_template('categories.html', categories=categories)
@app.route('/get_products')
def get_products():
# DB์์ ๋ชจ๋ Product ์กฐํ
products = Product.query.all()
return render_template('products.html', products=products)
@app.route('/get_users')
def get_users():
# DB์์ ๋ชจ๋ User ์กฐํ
users = User.query.all()
return render_template('users.html', users=users)
if __name__ == '__main__':
app.run(debug=True)
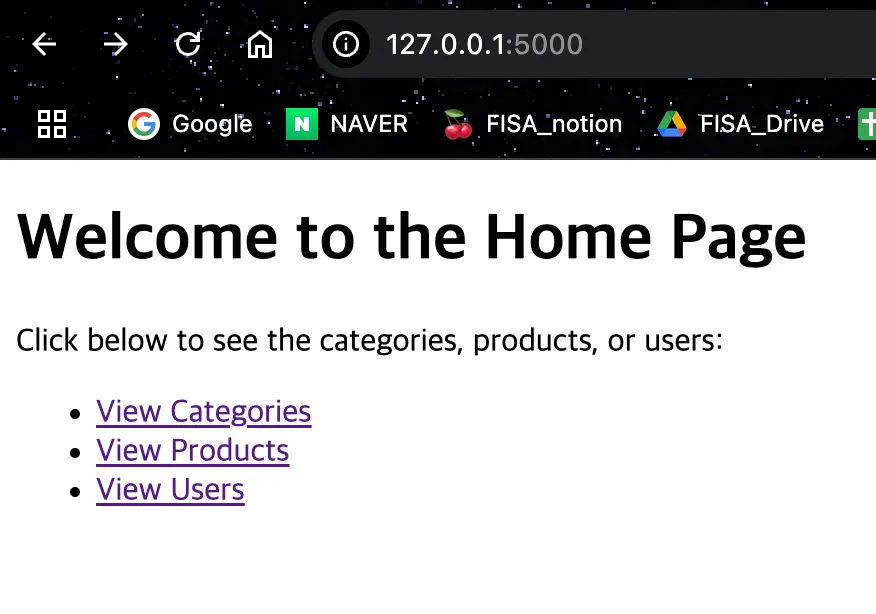
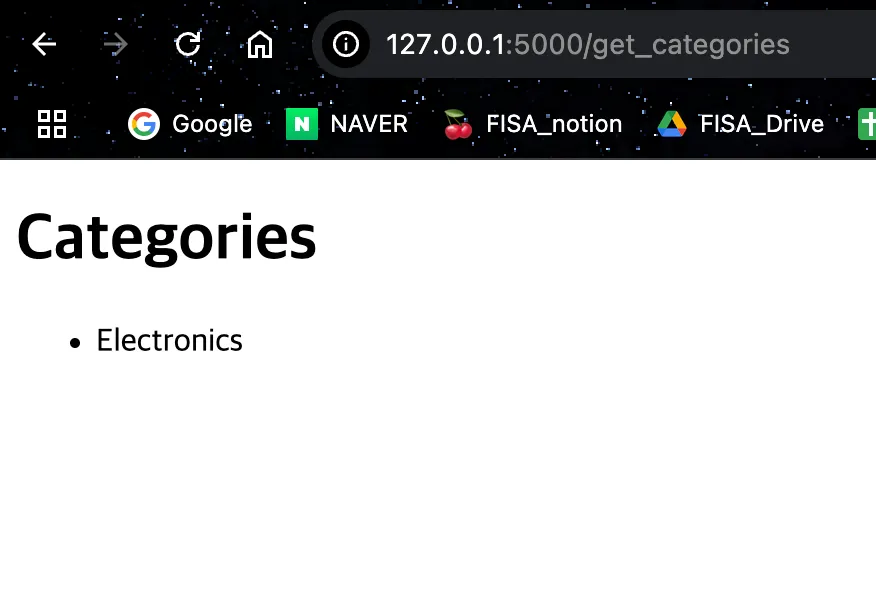
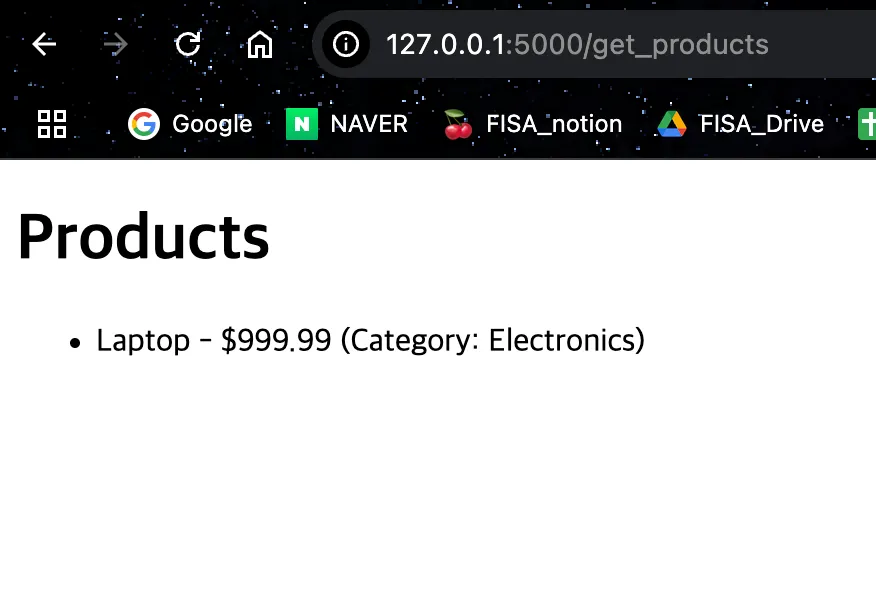
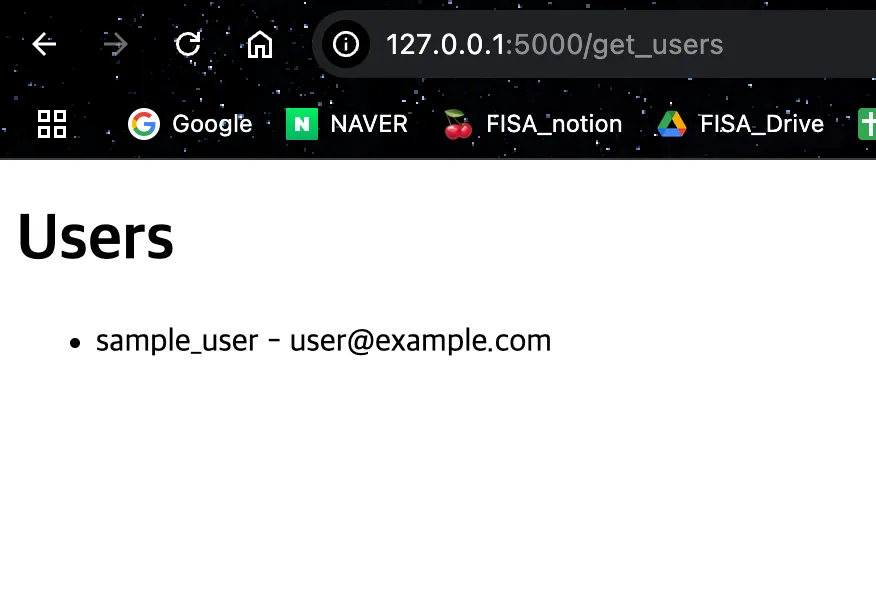
๊ธฐ์กด ๋ฐฉ์
- ๋ชจ๋ ๋ผ์ฐํธ์ ๊ธฐ๋ฅ์ด app.py ํ ๊ณณ์ ๋ชจ์ฌ ์์๊ณ ๋น์ฆ๋์ค ๋ก์ง(๋ฐ์ดํฐ๋ฒ ์ด์ค CRUD ์์ )๊ณผ ํ ํ๋ฆฟ ๋ ๋๋ง์ด ๋ชจ๋ ํผํฉ ๋จ
๋ณ๊ฒฝ๋ ๋ฐฉ์
- routes ํด๋: CRUD ์์
์ ๋ค๋ฃจ๋ ๋ผ์ฐํธ๋ฅผ ๊ด๋ฆฌํ๋ ํด๋
- ์ฌ๊ธฐ์ ์ฃผ๋ก API ๊ด๋ จ ๋ผ์ฐํธ๋ค์ด ์ ์ ๋จ
- ๋ฐ์ดํฐ๋ฒ ์ด์ค์ Product, Category, User ๋ชจ๋ธ์ ๋ํ CRUD
- ex) /api/get_products, /api/add_product, /api/update_category
- views ํด๋: ํ
ํ๋ฆฟ ๋ ๋๋ง๊ณผ ๊ด๋ จ๋ ๋ผ์ฐํธ๋ฅผ ๊ด๋ฆฌํ๋ ํด๋
- ์ฃผ๋ก GET ์์ฒญ์ ์ฒ๋ฆฌ, HTML ํ์ด์ง๋ฅผ ๋ ๋๋งํ๋ ์ญํ
- ๋น์ฆ๋์ค ๋ก์ง์ ์ฒ๋ฆฌํ์ง ์๊ณ ๋ฐ์ดํฐ๋ฅผ ํ ํ๋ฆฟ์ผ๋ก ์ ๋ฌํ์ฌ UI๋ฅผ ๋ณด์ฌ์ฃผ๋ ์ญํ
- ex) /get_categories (์นดํ ๊ณ ๋ฆฌ ๋ชฉ๋ก์ ์กฐํํ์ฌ HTML๋ก ๋ ๋๋ง)
https://github.com/seongjju/flask/tree/main/views
https://github.com/seongjju/flask/tree/main/routes
flask/routes at main ยท seongjju/flask
flask-practice. Contribute to seongjju/flask development by creating an account on GitHub.
github.com
'๐ผ ๋ฐฑ์ค๋ > Flask' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
flask with ORM (0) | 2025.03.27 |
---|---|
Flask ์น ์ ํ๋ฆฌ์ผ์ด์ ์ ๊ตฌ์กฐ์ ๋์ ์๋ฆฌ (0) | 2025.03.26 |
Flask๋ ํ ํ๋ฆฟ์ ์ฌ์ฉํ์ฌ ์น ํ์ด์ง๋ฅผ ๋์ ์ผ๋ก ๋ ๋๋งํ ์ ์๋ค.
ํ ํ๋ฆฟ์ HTML ํ์ผ๊ณผ ์ ์ฌํ์ง๋ง ๊ทธ ์์์ ํ์ด์ฌ ์ฝ๋(Jinja2 ํ ํ๋ฆฟ ์ธ์ด)๋ฅผ ์ฌ์ฉํ์ฌ ๋ฐ์ดํฐ๋ฅผ ๋์ ์ผ๋ก ์ฝ์ ํ๊ณ ์ ์ด
1. ํ ํ๋ฆฟ ํ์ผ ๋๋ ํ ๋ฆฌ ๊ตฌ์กฐ
/project_name
|-- app.py
|-- config.py
|-- models.py
|-- templates/
|-- categories.html
|-- products.html
|-- users.html
|-- test.db
templates/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page</h1>
<p>Click below to see the categories, products, or users:</p>
<ul>
<li><a href="/get_categories">View Categories</a></li>
<li><a href="/get_products">View Products</a></li>
<li><a href="/get_users">View Users</a></li>
</ul>
</body>
</html>
templates/categories.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Categories</title>
</head>
<body>
<h1>Categories</h1>
{% if categories %}
<ul>
{% for category in categories %}
<li>{{ category.name }}</li>
{% endfor %}
</ul>
{% else %}
<p>No categories available.</p>
{% endif %}
</body>
</html>
templates/products.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Products</title>
</head>
<body>
<h1>Products</h1>
{% if products %}
<ul>
{% for product in products %}
<li>{{ product.name }} - ${{ product.price }} (Category: {{ product.category.name }})</li>
{% endfor %}
</ul>
{% else %}
<p>No products available.</p>
{% endif %}
</body>
</html>
templates/users.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Users</title>
</head>
<body>
<h1>Users</h1>
{% if users %}
<ul>
{% for user in users %}
<li>{{ user.username }} - {{ user.email }}</li>
{% endfor %}
</ul>
{% else %}
<p>No users available.</p>
{% endif %}
</body>
</html>
app.py
from flask import Flask, jsonify, render_template
from models import db, Product, User, Category
import config
from flask_migrate import Migrate
app = Flask(__name__)
app.config.from_object(config)
# ORM ์ด๊ธฐํ
db.init_app(app)
# Flask-Migrate ์ด๊ธฐํ
migrate = Migrate(app, db)
@app.route('/')
def index():
# ๊ธฐ๋ณธ ๋ฉ์ธ ํ์ด์ง ๋ ๋๋ง
return render_template('index.html')
@app.route('/add_category')
def add_category():
# ์๋ก์ด Category ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
new_category = Category(name="Electronics")
db.session.add(new_category)
db.session.commit()
return "Category added!"
@app.route('/add_product')
def add_product():
# ์๋ก์ด Product ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
# ์ด๋ฏธ ์กด์ฌํ๋ Category ID๋ฅผ ํ ๋นํ์ฌ Product์ ์ฐ๊ฒฐ
category = Category.query.first() # ์ฒซ ๋ฒ์งธ ์นดํ
๊ณ ๋ฆฌ ๊ฐ์ ธ์ค๊ธฐ (์์๋ก)
new_product = Product(name="Laptop", price=999.99, category_id=category.id)
db.session.add(new_product)
db.session.commit()
return "Product added!"
@app.route('/add_user')
def add_user():
# ์๋ก์ด User ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ DB์ ์ถ๊ฐ
new_user = User(username="sample_user", email="user@example.com")
db.session.add(new_user)
db.session.commit()
return "User added!"
@app.route('/get_categories')
def get_categories():
# DB์์ ๋ชจ๋ Category ์กฐํ
categories = Category.query.all()
return render_template('categories.html', categories=categories)
@app.route('/get_products')
def get_products():
# DB์์ ๋ชจ๋ Product ์กฐํ
products = Product.query.all()
return render_template('products.html', products=products)
@app.route('/get_users')
def get_users():
# DB์์ ๋ชจ๋ User ์กฐํ
users = User.query.all()
return render_template('users.html', users=users)
if __name__ == '__main__':
app.run(debug=True)
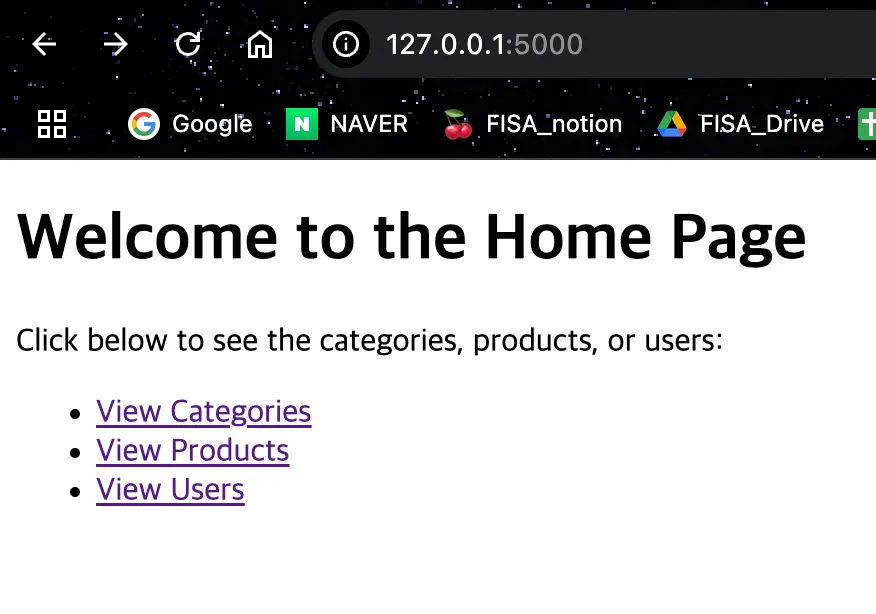
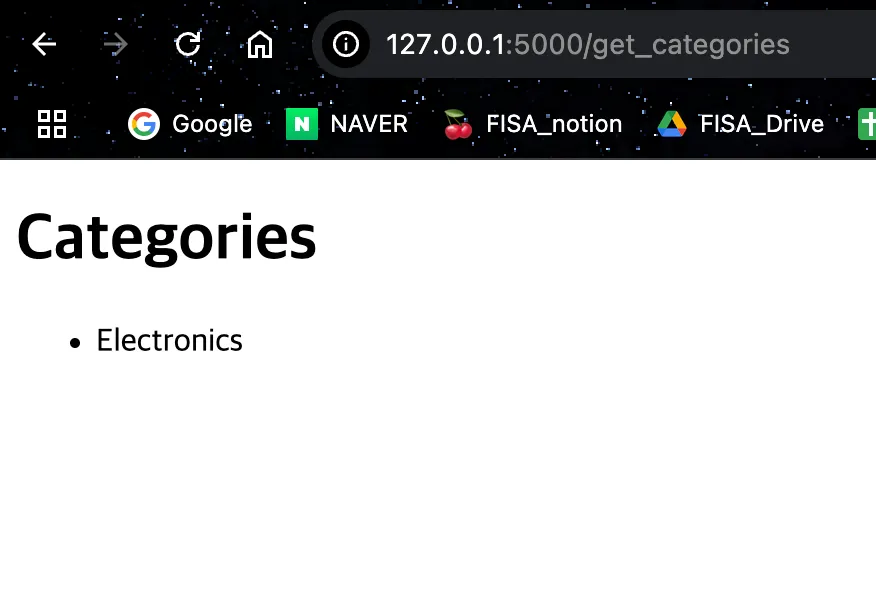
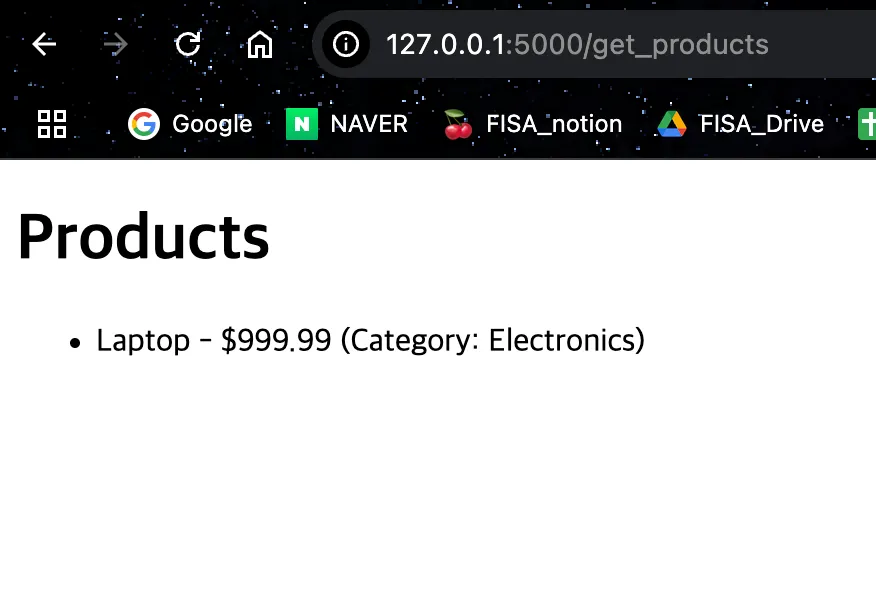
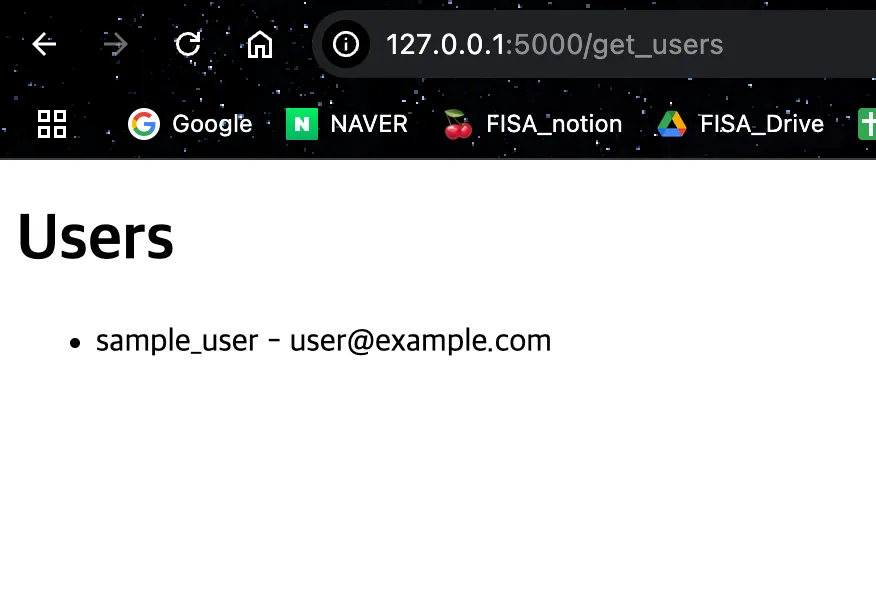
๊ธฐ์กด ๋ฐฉ์
- ๋ชจ๋ ๋ผ์ฐํธ์ ๊ธฐ๋ฅ์ด app.py ํ ๊ณณ์ ๋ชจ์ฌ ์์๊ณ ๋น์ฆ๋์ค ๋ก์ง(๋ฐ์ดํฐ๋ฒ ์ด์ค CRUD ์์ )๊ณผ ํ ํ๋ฆฟ ๋ ๋๋ง์ด ๋ชจ๋ ํผํฉ ๋จ
๋ณ๊ฒฝ๋ ๋ฐฉ์
- routes ํด๋: CRUD ์์
์ ๋ค๋ฃจ๋ ๋ผ์ฐํธ๋ฅผ ๊ด๋ฆฌํ๋ ํด๋
- ์ฌ๊ธฐ์ ์ฃผ๋ก API ๊ด๋ จ ๋ผ์ฐํธ๋ค์ด ์ ์ ๋จ
- ๋ฐ์ดํฐ๋ฒ ์ด์ค์ Product, Category, User ๋ชจ๋ธ์ ๋ํ CRUD
- ex) /api/get_products, /api/add_product, /api/update_category
- views ํด๋: ํ
ํ๋ฆฟ ๋ ๋๋ง๊ณผ ๊ด๋ จ๋ ๋ผ์ฐํธ๋ฅผ ๊ด๋ฆฌํ๋ ํด๋
- ์ฃผ๋ก GET ์์ฒญ์ ์ฒ๋ฆฌ, HTML ํ์ด์ง๋ฅผ ๋ ๋๋งํ๋ ์ญํ
- ๋น์ฆ๋์ค ๋ก์ง์ ์ฒ๋ฆฌํ์ง ์๊ณ ๋ฐ์ดํฐ๋ฅผ ํ ํ๋ฆฟ์ผ๋ก ์ ๋ฌํ์ฌ UI๋ฅผ ๋ณด์ฌ์ฃผ๋ ์ญํ
- ex) /get_categories (์นดํ ๊ณ ๋ฆฌ ๋ชฉ๋ก์ ์กฐํํ์ฌ HTML๋ก ๋ ๋๋ง)
https://github.com/seongjju/flask/tree/main/views
https://github.com/seongjju/flask/tree/main/routes
flask/routes at main ยท seongjju/flask
flask-practice. Contribute to seongjju/flask development by creating an account on GitHub.
github.com
'๐ผ ๋ฐฑ์ค๋ > Flask' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
flask with ORM (0) | 2025.03.27 |
---|---|
Flask ์น ์ ํ๋ฆฌ์ผ์ด์ ์ ๊ตฌ์กฐ์ ๋์ ์๋ฆฌ (0) | 2025.03.26 |